Factory design pattern Java Factory pattern tutorial by Erwan LE TUTOUR Javarevisited
Table Of Content
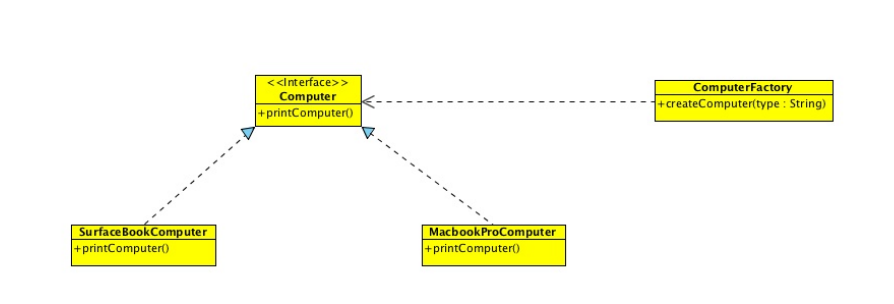
It uses the accountType to create the corresponding type of BankAccount. In this example, I’ll create a simple “dog factory” that can return a variety of Dog types, where the “Dog” that is returned matches the criteria I specify. For instance, I might tell the factory that I want a small dog, or a large dog, and the dog factory will give me a dog of the type I asked for. Probably the most obvious and convenient place where this code could be placed is the constructor of the class whose objects we’re trying to reuse. However, a constructor must always return new objects by definition. Car is parent class of all car instances and it will also contain the common logic applicable in car making of all types.
Factory Design Pattern Implementation
Software systems undergo constant changes and they are unstable. We must consider designing where the objects and relationships are loosely coupled. Over the course of this article, we will examine one of the most commonly used patterns, the Factory method pattern in java.
Factory Method Java Design Patterns
Each object is created through a factory method available in the factory - which can either be an interface or an abstract class. The Factory Method Pattern (also known as the Virtual Constructor or Factory Template Pattern) is a creational design pattern used in object-oriented languages. These are the actual implementations of the Currency interface. Each concrete product provides its own implementation of the getSymbol() method.
Build a Hibernate SessionFactory by example - TheServerSide.com
Build a Hibernate SessionFactory by example.
Posted: Sun, 02 Aug 2020 07:00:00 GMT [source]
Creational Software Design Patterns in Java
Note that this pattern is also known as Factory Method Design Pattern. In factory design pattern we create an object from a factory class without exposing the creation logic to the client. The type of the object will be one of the subclasses and it will depends upon the input which we pass to the factory. The factory design pattern offers a solution for efficient object creation in software development. It reduces coupling between client code and object construction code and results in maintainable, scalable applications. Understanding when and how to apply the factory design pattern effectively can enhance your skills as a software developer and improve your applications.
Concrete Creators
And as you’re about to see, the signature of the factory method shows that it will be returning a class which implements my base class, in this case, my Dog interface. Spice Digital Inc. company now decided to make their global presence. They want to extend their business to London and NewYork location. Looking at the market interest, London factory will produce only SpiceBolt and SpiceFire model and New York will produce SpiceFire and SpicePlus. Let us have a glance at the below class diagram that using the Factory method design pattern. For example, to add a new product type to the app, you’ll only need to create a new creator subclass and override the factory method in it.
After finishing this article, while writing your application, consider using the Java factory pattern. Factory Method Design Pattern define an interface for creating an object, but let subclass decide which class to instantiate. As you can see, the creation of objects in the method create(AccountType type) depends on the constructor of the sub-classes. Therefore, if the constructor has many parameters, the factory method can become complex. The problem with this type of code is that one class – drinkCafe, depends on all the subclasses that are being created inside of it.
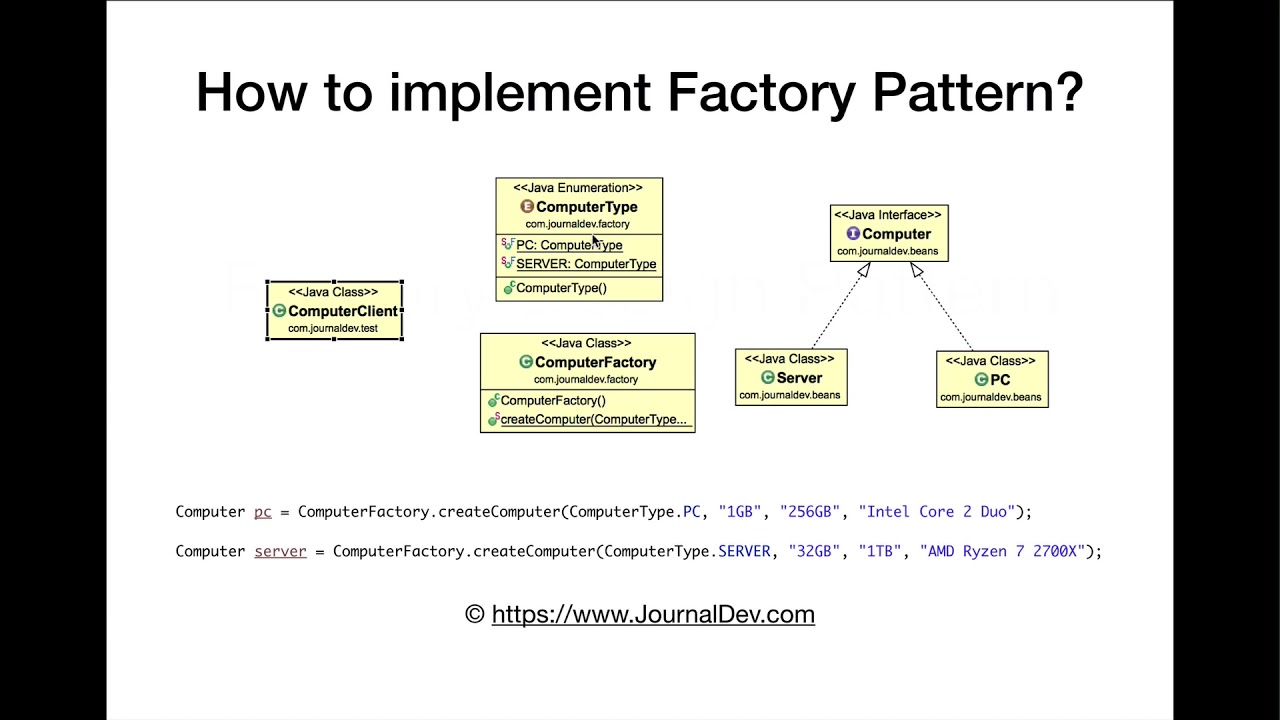
You often experience this need when dealing with large, resource-intensive objects such as database connections, file systems, and network resources. Javatpoint provides tutorials with examples, code snippets, and practical insights, making it suitable for both beginners and experienced developers. Use the Factory Method when you want to provide users of your library or framework with a way to extend its internal components. In this example, Buttons play a product role and dialogs act as creators. Factory Pattern is one of the Creational Design pattern and it’s widely used in JDK as well as frameworks like Spring and Struts.

So far we learned what is Factory method design pattern and how to implement it. I believe now we have a fair understanding of the advantage of this design mechanism. Factory methods pervade toolkits and frameworks.The preceding document example is a typical use in MacApp and ET++. AccountFactory is a class that implements the Factory design pattern.
Then, using a SpaceshipFactory as our communication point with these, we'll instantiate objects of Spaceship type, though, implemented as concrete classes. This logic will stay hidden from the end user, as we'll be specifying which implementation we want through the argument passed to the SpaceshipFactory method used for instantiation. If the factory is a class - the subclasses can use existing implementation or optionally override factory methods.
Therefore, this design pattern falls in the Creational category of design patterns. There are other patterns also that fall in this category, such as the Singleton Design Factory. The factory design pattern says that code should be open for extension but closed for modification, as all patterns agree to that. A Factory Pattern or Factory Method Pattern says that just define an interface or abstract class for creating an object but let the subclasses decide which class to instantiate.
The Factory Method defines a method, which should be used for creating objects instead of using a direct constructor call (new operator). Subclasses can override this method to change the class of objects that will be created. Factory pattern introduces loose coupling between classes which is the most important principle one should consider and apply while designing the application architecture. Loose coupling can be introduced in application architecture by programming against abstract entities rather than concrete implementations. This not only makes our architecture more flexible but also less fragile. If object creation code is spread in the whole application, and if you need to change the process of object creation then you need to go in each and every place to make necessary changes.
Use the Factory Method when you don’t know beforehand the exact types and dependencies of the objects your code should work with. This example illustrates how the Factory Method can be used for creating cross-platform UI elements without coupling the client code to concrete UI classes. Subclasses can alter the class of objects being returned by the factory method.
Comments
Post a Comment